Enumeration Support
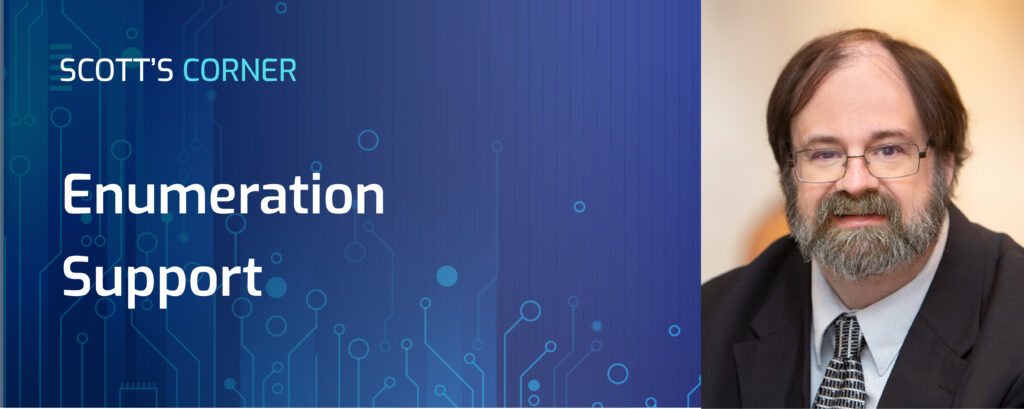
Bad Joke of the Month:
Yesterday, I ate a clock. It was very time consuming…especially when I went back for seconds.
Enumeration Support
On October 10, IBM announced the latest RPG features. Among the new features were Enumerations (sometimes called ‘mnemonics’) – which is a group of named constants meant to identify all of the codes available for a specific field’s value.
A simple example might be marital status:
dcl-enum maritalStatus;
single ‘S’;
married ‘M’;
separated ‘E’;
divorced ‘D’;
widowed ‘W’;
end-enum;
Think of each line of this as a named constant. You can use them the same way that you’d use a constant. For example, prior to the enumeration feature, if you wanted to check if an employee was divorced, you might write code like the following:
if emprec.mstatus = ‘D’;
// code for a divorced employee
endif;
Since an enum can be used like a named constant, you can check using the constant value instead:
if emprec.mstatus = divorced;
// code for a divorced employee
endif;
This benefits programmers reading the source code who may not know what the different marital status codes might be.
Enumerations can also be qualified by adding the qualified keyword to the dcl-enum line. It works very much like a data structure. When you qualify them, each value (aka named constant) must be prefixed by the enumeration name, followed by a dot, and then the value name. This is helpful because each time you see a value, you will know immediately that it’s part of that particular enumeration.
dcl-enum maritalStatus qualified;
single ‘S’;
married ‘M’;
separated ‘E’;
divorced ‘D’;
widowed ‘W’;
end-enum;
if emprec.mstatus = maritalStatus.divorced;
// code for a divorced employee
endif;
As you might expect, you can use each value as an option in a SELECT/WHEN statement if you wanted to, for example, print a descriptive name for a marital status.
select emprec.mstatus;
when-is maritalStatus.single;
desc = ‘single’;
when-is maritalStatus.married;
desc = ‘married’;
when-is maritalStatus.separated;
desc = ‘separated’;
when-is maritalStatus.divorced;
desc = ‘divorced’;
when-is maritalStatus.widowed;
desc = ‘widowed’;
other;
desc = ‘invalid’;
endsl;
Since enumerations are a list of values, they can also be used the same way you might use the %LIST built-in function. For example, suppose you have an interactive program where a user keys a marital status code on the screen, and you want to see if it’s valid. The older way might be to use the %LIST built-in function as follows:
if emprec.mstatus in %LIST(‘S’: ‘M’: ‘E’: ‘D’: ‘W’);
// valid code
endif;
Now you can do the same thing by checking if the value is in the enumeration directly:
if emprec.mstatus in maritalStatus;
// valid code
endif;
Since the SELECT/WHEN-IN opcode works with lists, you can also use enumerations with SELECT/WHEN-IN very similarly to the way you would use them with the in keyword of an if statement.
select emprec.mstatus;
when-in maritalStatus;
// valid status
when-is ‘ ‘;
// blank = unspecified
other;
// unexpected code.
endsl;
Since you can loop through the items in a list, you can loop through all of the values of an enum with FOR-EACH.
for-each code in maritalStatus;
dsply code;
endfor;
It’s also very easy to assign the codes to an array (though,
I’m having a hard time thinking of use cases for this.)
dcl-s array char(1) dim(5);
array = maritalStatus;
for-each code in array;
dsply code;
endfor;
My example has been for one letter codes, but it doesn’t have to be. Each value can be any data type (string, number, date, time, and so forth.)
You can learn more, and find the PTFs needed to install enumeration support on your 7.4 or 7.5 machine at the following link: https://ibm.biz/rpgcafe_fall_2023_enums
As I’ve shown, the new enumeration support is very versatile, and will make your code easier to read, easier to understand, and even easier to write. Give it a try!